HttpsUtil
放出个常用的访问hppts的工具类。该工具类共两个类文件,一个用于实现证书的java类,一个用于实际调用的java类。
MyX509TrustManager
用于创建访问https时所需要的证书。 [toggle hide=“no” title=“MyX509TrustManager” color=“red”]
import javax.net.ssl.X509TrustManager;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
public class MyX509TrustManager implements X509TrustManager
{
public void checkClientTrusted(X509Certificate[] chain, String authType)
throws CertificateException
{}
public void checkServerTrusted(X509Certificate[] chain, String authType)
throws CertificateException
{}
public X509Certificate[] getAcceptedIssuers()
{
return null;
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
[/toggle]
HttpsUtil
用于项目中实际调用 [toggle hide=“no” title=“HttpsUtil” color=“red”]
import org.json.JSONObject;
import sun.net.www.protocol.https.HttpsURLConnectionImpl;
import javax.net.ssl.SSLContext;
import javax.net.ssl.TrustManager;
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.ConnectException;
import java.net.URL;
import java.security.SecureRandom;
public class HttpsUtil {
/**
* GET/POST方式请求服务器(https协议)
*
* @param requestUrl
* 请求地址
* @param requestMethod
* 请求类型(GET/POST)
* @param outputStr
* 参数
* @return JSONObject
*
* */
public static JSONObject httpRequest(String requestUrl, String requestMethod, String outputStr)
{
JSONObject jsonObject = new JSONObject();
StringBuffer buffer = new StringBuffer();
try
{
TrustManager[] tm = { new MyX509TrustManager() };
SSLContext sslContext = SSLContext.getInstance("SSL", "SunJSSE");
sslContext.init(null, tm, new SecureRandom());
// SSLSocketFactory ssf = sslContext.getSocketFactory();
URL url = new URL(requestUrl);
HttpsURLConnectionImpl httpUrlConn = (HttpsURLConnectionImpl)url
.openConnection();
httpUrlConn.setDoOutput(true);
httpUrlConn.setDoInput(true);
httpUrlConn.setUseCaches(false);
httpUrlConn.setRequestMethod(requestMethod);
if ("GET".equalsIgnoreCase(requestMethod)) {
httpUrlConn.connect();
}
if (outputStr != null)
{
OutputStream outputStream = httpUrlConn.getOutputStream();
outputStream.write(outputStr.getBytes("UTF-8"));
outputStream.close();
}
InputStream inputStream = httpUrlConn.getInputStream();
InputStreamReader inputStreamReader = new InputStreamReader(
inputStream, "utf-8");
BufferedReader bufferedReader = new BufferedReader(
inputStreamReader);
String str = null;
while ((str = bufferedReader.readLine()) != null) {
buffer.append(str);
}
bufferedReader.close();
inputStreamReader.close();
inputStream.close();
inputStream = null;
httpUrlConn.disconnect();
System.out.println("buffer:"+buffer);
jsonObject.put("data",buffer.toString());
System.out.println("jsonObject:"+jsonObject);
}
catch (ConnectException ce)
{
ce.printStackTrace();
}
catch (Exception e)
{
e.printStackTrace();
}
return jsonObject;
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
[/toggle]
调用方式
先给出两种调用方式,更多组合请自行百搭
JSONObject result = HttpsUtil.httpRequest(url, "POST", prm); JSONObject result = HttpsUtil.httpRequest(url+prm, "GET", “”);
1
2
预览
除特别注明外,本站所有文章均为 windcoder 原创,转载请注明出处来自: httpsutil
Loading comments...
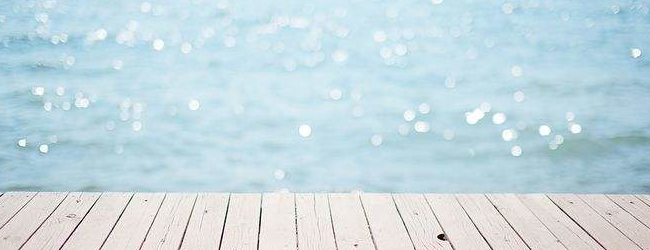
预览
暂无数据