Java学Go入门笔记02-切片
该系列是在有Java基础上学习Go语言的一些基础的对比学习笔记。
切片(Slice)
Go 中更常用的不是数组而是切片(Slice),即动态数组,长度不固定,即将满时自动扩容。
- 切片是对数组一个连续片段的引用,数组定义中不指定长度即为切片
- 未初始化之前默认是
nil
,长度为0 - 使用
[(start):(end)]
截取部分切片,start
和end
分别时开始位置和结束位置,均可选。如:[1:3]
、[:]
- 使用
append()
追加元素 - 删除 index 处时元素,使用
append(slice[:index],slice[index+1:]...)
语法
简单写下声明语句
var identifier []type
Make 和 New
new
返回指针地址make
返回第一个元素,可预设内存空间,避免未来的内存拷贝
// 返回指针
mySlice1 := new([]int)
// 返回第一个元素
mySlice2 := make([]int, 0)
mySlice3 := make([]int, 10)
mySlice4 := make([]int, 10, 20)
2
3
4
5
6
切片常见问题
切片相互赋值
- 内置函数
append
将元素追加到切片末尾。 - 当切片有足够容量时,自动追加到当前切片的底层数组中
- 当切片容量不足时,将分配新的底层数组,并将元素追加进新数组,最终返回新数组
The append built-in function appends elements to the end of a slice. If it has sufficient capacity, the destination is resliced to accommodate the new elements. If it does not, a new underlying array will be allocated. Append returns the updated slice. It is therefore necessary to store the result of append, often in the variable holding the slice itself:
slice = append(slice, elem1, elem2) slice = append(slice, anotherSlice...)
1
2As a special case, it is legal to append a string to a byte slice, like this:
slice = append([]byte("hello "), "world"...)
1
func append(slice []Type, elems ...Type) []Type
a:=[]int{}
b:=[]int{1,2,3}
c:=a
// 由于值传递,a的追加修改永远不会影响到c
a=append(b,1)
// 长度3,初始容量3
a1:=make([]int,3,3)
2
3
4
5
6
7
copy
- 用法:
copy(arry1, arry2)
- arry1目标切片,arry2 源切片
- 将源切片 arry2 复制到目标切片 arry1 ,复制长度以两者中最小的为准:即arry1长度最小时,将 arry2 中长度为 arry1 长度的数据复制到 arry1,反之将 arry1 中 arry2 长度的数据全复制到为 arry2 的。两个 slice 指向同⼀底层数组,直接覆盖对应位置。
The copy built-in function copies elements from a source slice into a destination slice. (As a special case, it also will copy bytes from a string to a slice of bytes.) The source and destination may overlap. Copy returns the number of elements copied, which will be the minimum of len(src) and len(dst).
func copy(dst, src []Type) int
For循环中的切片值修改
// ArrayObj 是一个结构体( struct )
type ArrayObj struct {
I int
}
// 如此只改变value 值,无法改变切片实际值
mySlice4 :=[]entity.ArrayObj{{1},{2},{3}}
for _,value:= range mySlice4 {
value.I *=2
}
fmt.Println(mySlice4)
// 若想改变,需要通过下标索引
for index,_:= range mySlice4 {
mySlice4[index].I *=2
}
fmt.Println(mySlice4)
2
3
4
5
6
7
8
9
10
11
12
13
14
15
除特别注明外,本站所有文章均为 竹风清语 原创,转载请注明出处来自: javaxuegorumenbiji02-qiepian
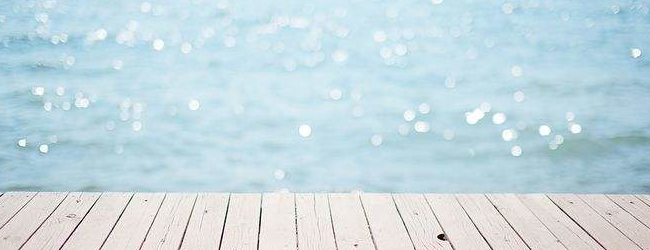
暂无数据