Python第五周学习笔记
前言
发现之前并没有字符串相关的介绍,再者此次做的有点多,就整理一下发出来算了。此处的例子将代码和运行结果写在了一起,并未分开,细心看下也是可以看懂的,一般不存在 >>>的行均为结果。
正文
长度(len()函数)
例:
>>> a = 'Michael'
>>> len(a)
7
2
3
例2:
>>> a="hello woird"
>>> range(len(a))
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
2
3
拼接(+)
只可拼接字符/串,不可拼接整数 例:
>>> name = a +' Jordan'
>>> print name
Michael Jordan
2
3
重复(*)
必须×整数 例:
>>> name * 3
'Michael JordanMichael JordanMichael Jordan'
2
成员运算符(in)
判断一个字符串是否是另一个字符串的字串,区分大小写 返回值:True或False 例:
>>> 'a' in name
True
>>> 'jordan' in name
False
>>> 'Jordan' in name
True
2
3
4
5
6
for语句
枚举字符串的每个字符 例:
for>>> for c in name:
print c
M
i
c
h
a
e
l
J
o
r
d
a
n
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
字符串索引(index)
字符串中的每个字符都有一个索引值(下标) 索引从0(向前)或-1(向后)开始
>>> s = 'Hello World'
>>> s[-1]
'd'
>>> s[2]
'l'
>>> s[-2]
'l'
>>> s[-3]
'r'
2
3
4
5
6
7
8
9
[:]切片
选择字符串的子序列
1.[star:finish]
star:子序列开始位置的索引值 finish:子序列结束位置的下一个字符的索引值(即不包含finish位置的值) 例:
>>> s = 'Hello World'
>>> s[6:10]
'Worl'
>>> s[6:]
'World'
>>> s[:6]
'Hello '
#两个都不写为整个字符串
>>> s[:]
'Hello World'
#finish可超出字符串范围,此时不提时越界,而是从字符串开始到结尾
>>> s[:20]
'Hello World' <br>
2
3
4
5
6
7
8
9
10
11
12
13
2.接受三个参数[start:finish:countBy]
计数参数:默认countBy为1 例:
>>> s = 'Hello World'
>>> s[4:10:2]
'oWr' <br>
2
3
字符串是不可改变的
一旦生成,内容不能改变 例:
>>> s = 'Hello World'
>>> s[1] = 'a'
2
结果报如下错误: [callout class=“danger” title=“”] Traceback (most recent call last): File “<pyshell#25>”, line 1, in <module> s[1] = ‘a’ TypeError: ‘str’ object does not support item assignment [/callout]
通过切片等操作,生成一个新的字符串
例:
>>> d =s
>>> d = d[:1]+'a'+s[2:]
>>> d
'Hallo World'
>>> d[:1]+'o'+s[2:]
'Hollo World'
>>> d
'Hallo World'
2
3
4
5
6
7
8
字符串方法
replace(old,new)
生成一个新的字符串,其中使用new替换old子串 依旧无法修改原字符串,若想修改需要将其重新赋值给原字符串
find()
查询其字串,返回第一次出现的时的下标。 例:
>>> d = 'Hello World'
>>> d.find("l")
2
2
3
split()
将原字符串切分成若干字符串,若任何参数,则在空格处切分。 例:
>>> s = 'hello world'
>>> s.split()
['hello', 'world']
2
3
str.strip()
去开始的空格回车等字符 例:
>>> s=" Hello word"
>>> s
' Hello word'
>>> s.strip()
'Hello word'
2
3
4
5
str.title()
首字母大写,其余字母小写 例:
>>> s = 'hello world'
>>> s.title()
'Hello World'
2
3
str.isdigit()
判断这个字符是否是数字字符 即’0’~‘9’ 例:
>>> s = 'hello world'
>>> s.isdigit()
False
2
3
s.ljust(width,[fillchar])
s.ljust(width,[fillchar]) 左对齐输出15个字符,不足部分用’.'补足,默认用空格补足 例:
>>> s.ljust(15,'.')
'hello world....'
2
3
s.rjust(width,[fillchar])
s.rjust(width,[fillchar]) 右对齐输出15个字符,不足部分用’[fillchar]'补足,默认用空格补足 例:
>>> s.rjust(15)
' hello world'
2
s.count([fillchar])
获取字符串中第一个[fillchar]的下标 例:
>>> s = 'hello world'
>>> s.count('l')
3
2
3
字符串比较
字典序: [callout class=“danger” title=“”] 首先比较两个字符串的第一个字符 若相同,则比较下一个字符 若不同,则字符串的大小关系由这两个字符的关系决定 若其中一个字符为空(较短),则其更小 [/callout]
字符串格式化
format方法
>>> print 'hello {} good {}.'.format(5,'DAY')
hello 5 good DAY.
2
括号的格式 [callout class=“danger” title=“”] {field name:align width.precision type} {域的名字:对齐方式 占用的宽度.精度 类型} >(大于号)右对齐 <(小于号)左对齐 [/callout] 例:
>>> import math
>>> "Pi is {}".format(math.pi)
'Pi is 3.14159265359'
>>> 'PI is {:.4f}'.format(math.pi)
'PI is 3.1416'
>>> 'PI is {:.4e}'.format(math.pi)
'PI is 3.1416e+00'
>>> 'PI is {:9.4f}'.format(math.pi)
'PI is 3.1416'
>>> 'PI is {:<9.4f}'.format(math.pi)
'PI is 3.1416 '
2
3
4
5
6
7
8
9
10
11
文件操作步骤
1.打开文件
f= open(filename,mode)
mode有r(读,默认),w(写)等
2.按行读取文件内容
for line in f:
pass
2
3.关闭文件
f.close()
写文件
f.write(str)
正则表达式
用来描述字符串的模式: .表示任意字符 d+表示一系列数字 [a-z]表示一个小写字母
待写专项
正则表达式 字符串处理函数
除特别注明外,本站所有文章均为 windcoder 原创,转载请注明出处来自: python-di-wu-zhou-xue-xi-bi-ji
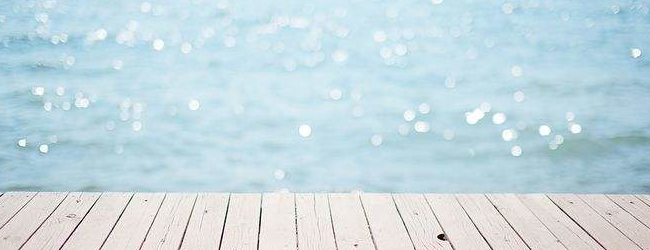
用Django写个网站玩玩更有意思~
有点长啊
回复 @站长工具: 基本上是课堂笔记了= =
YUSI2.1主题发布了,不要错过哦 http://tmy123.com/Yusi2.1.html
回复 @同盟源: 谢谢提醒,没想到那款主题到你那了,而且变的相当漂亮和强大
好好学吧~
还记得小时候,上课做的笔记。
看着这些好有成就感!
没事逛逛。
回复 @王语双: 感谢还记得O(∩_∩)O~
感觉直接开始写脚本学得比较快,基础折腾来折腾去就是这些东西
回复 @恋羽: 赞同,感觉学多少次基础都比不上一个实践项目记得深,一语点醒梦中人啊,我说怎么总觉得缺了点什么,太感谢了