TensorFlow入门:一篇机器学习教程
前言
这周又送来一篇python实现的机器学习的入门文章。一起学习吧。
原文:Getting Started with TensorFlow: A Machine Learning Tutorial 作者: DINO CAUSEVIC
正文
TensorFlow是一个由Google创建的开源软件库,用于实现机器学习和深度学习系统。这两个名称包含一系列强大的算法,它们共享一个共同的挑战——让计算机学习如何自动识别复杂模式和/或做出最佳决策。 如果你对这些系统的细节感兴趣,你可以从Toptal博客文章中了解更多有关机器学习和深度学习的内容。 TensorFlow的核心是数据流编程库。它利用各种优化技术使数学表达式的计算更容易和更高效。 TensorFlow的一些关键特性是:
- 有效地处理涉及多维数组的数学表达式
- 深度神经网络和机器学习概念的良好支持
- GPU / CPU计算,可以在两种架构上执行相同的代码
- 跨计算机和庞大的数据集计算的高可扩展性
总之,这些功能使TensorFlow成为生产规模下机器智能的完美框架。 在这个TensorFlow教程中,您将学习如何在TensorFlow中使用简单而强大的机器学习方法,以及如何使用它的一些辅助库来调试,可视化和调整使用它创建的模型。
安装TensorFlow
我们将使用TensorFlow Python API,它适用于Python 2.7和Python 3.3+。GPU版本(仅Linux)需要Cuda Toolkit 7.0+和cuDNN v2 +。 我们将使用Conda软件包依赖管理系统来安装TensorFlow。Conda允许我们在机器上分离多个环境。您可以从这里学习如何安装Conda 。 在安装了Conda之后,我们可以创建用于安装和使用TensorFlow的环境。下面的命令将创建我们的环境,其中有一些类似NumPy的额外库,一旦我们开始使用TensorFlow,这将非常有用。 在这个环境中安装的Python版本是2.7,我们将在这篇文章中使用这个版本。
conda create --name TensorflowEnv biopython
为了让事情变得容易,我们在这里安装biopython而不是NumPy。这包括NumPy和我们将需要的其他一些软件包。您可以随时根据需要使用conda install
或pip install
命令来安装软件包。 以下命令将激活创建的Conda环境。我们将能够使用其中安装的软件包,而无需混用全局或其他环境中安装的软件包。
source activate TensorFlowEnv
pip安装工具是Conda环境的标准组成部分。我们将使用它来安装TensorFlow库。在此之前,一个好的第一步是使用以下命令将pip更新为最新版本:
pip install --upgrade pip
现在我们将通过运行如下命令安装TensorFlow:
pip install tensorflow
TensorFlow的下载和构建可能需要几分钟的时间。在写这篇文章的时候,它安装的是TensorFlow 1.1.0。
数据流图
在TensorFlow中,使用数据流图来描述计算。图的每个节点表示数学运算的实例(如加法,除法或乘法),每个边是执行操作的多维数据集(张量)。 当TensorFlow与计算图形一起工作时,它们被管理在每个节点代表一个操作实例化的地方,每个操作都有0个或更多的输入和0或更多的输出。 TensorFlow中的边可以分为两类:正常边传输数据结构(张量),其中一个操作的输出可能成为另一个操作的输入,而特殊边则用于控制两个节点之间的依赖关系来设置一个节点等待另一个节点完成的操作顺序。
简单的表达式
在我们继续讨TensorFlow的元素之前,我们首先要做一个与TensorFlow有关的工作,来了解TensorFlow项目是什么样子的。 让我们从简单的表达式开始,并假设出于某种原因,我们想要在TensorFlow fashion中评估函数y = 5*x + 13。 在简单的Python代码中,它看起来像:
x = -2.0
y = 5*x + 13
print y
2
3
在这个例子中,我们得到了3.0下的结果。 现在我们将上面的表达式转换成 TensorFlow terms。
常量
在TensorFlow中,使用具有签名constant(value, dtype=None, shape=None, name=‘Const’, verify_shape=False)的函数常量来创建常量,其中value
是将在进一步计算中使用的实际常数值,dtype
是数据类型参数(例如, float32/64, int8/16等),shape
是可选的尺寸,name
是张量的可选名称,最后一个参数是一个布尔值,表示是否验证值的形状。 如果您需要在训练模型中使用具有特定值的常量,则constant
可以按照以下示例使用该对象:
z = tf.constant(5.2, name="x", dtype=tf.float32)
变量
TensorFlow中的变量是包含张量的内存缓冲区,它们必须被显式初始化并在图中使用才能在会话中保持状态。通过简单地调用构造函数,就可以在计算图中添加变量。 一旦从训练模型开始,变量就特别有用,它们被用来保存和更新参数。作为构造函数参数传递的初始值表示可作为张量转换或返回的张量或对象。这意味着如果我们想用一些预定义的或随机的值来填充一个变量,然后在训练过程中使用这个值并且在迭代中更新,我们可以用下面的方式来定义它:
k = tf.Variable(tf.zeros([1]), name="k")
在TensorFlow中使用变量的另一种方法是在计算中,该变量不可训练,并且可以通过以下方式进行定义:
k = tf.Variable(tf.add(a, b), trainable=False)
Sessions
为了实际评估节点,我们必须在session中运行一个计算图。 Session封装了TensorFlow运行时的控制和状态。没有参数的session将使用在当前session中创建的默认图形,否则session类接受在该会话中使用的图形参数来执行。 下面是一个简短的代码片段,显示了如何在TensorFlow中使用上面定义的术语来计算一个简单的线性函数。
import tensorflow as tf
x = tf.constant(-2.0, name="x", dtype=tf.float32)
a = tf.constant(5.0, name="a", dtype=tf.float32)
b = tf.constant(13.0, name="b", dtype=tf.float32)
y = tf.Variable(tf.add(tf.multiply(a, x), b))
init = tf.global_variables_initializer()
with tf.Session() as session:
session.run(init)
print session.run(y)
2
3
4
5
6
7
8
9
10
11
12
13
使用TensorFlow:定义计算图
使用数据流图的好处在于,执行模型与其执行位置(在CPU,GPU或某种组合上)是分开的,一旦实现,TensorFlow中的软件可以在CPU或GPU上使用,其中隐藏了所有与代码执行相关的复杂性。 可以在使用TensorFlow库的过程中构建计算图,而不必显式实例化Graph对象。 TensorFlow中的Graph对象可以通过简单的代码行来创建c = tf.add(a, b)
。这将创建一个操作节点,它需要两个张量a
和b
并将其和c
作为输出。 计算图是一个使用库的内置过程,不需要直接调用图对象。TensorFlow中的一个图形对象,包含一组操作和张量作为数据单元,用于允许同一个进程并包含多个图的操作之间,其中每个图将被分配给不同的session。例如,代码的简单线c = tf.add(a, b)
将创建需要两个张量的操作的节点a
和b
作为输入,并产生它们的总和c
作为输出。 TensorFlow还提供了一个feed机制,用于将张量修补到图中的任何操作,其中feed将用张量值替换操作的输出。供稿数据作为run()
函数调用的参数传递。 占位符是TensorFlow允许开发人员通过绑定在某些表达式中的占位符将数据注入到计算图中的方式。占位符的签名是:
placeholder(dtype, shape=None, name=None)
其中dtype是张量中的元素的类型,并且可以提供要被feed的张量的shape和操作的名称。 如果形状不合格,张量可以以任何形状进给。一个重要的注意事项是占位符张量必须被提供数据,否则,在执行会话时,如果缺少该部分,则占位符将生成以下结构的错误:
InvalidArgumentError (see above for traceback): You must feed a value for placeholder tensor 'y' with dtype float
占位符的优势在于它允许开发人员创建操作,并且通常可以创建计算图形,而无需事先为其提供数据,并且数据可以从运行时从外部源添加。 我们来看一个简单的乘法两个整数x
和y
TensorFlow方式的问题,其中一个占位符将通过会话run
方法与一个提要机制一起使用。
import tensorflow as tf
x = tf.placeholder(tf.float32, name="x")
y = tf.placeholder(tf.float32, name="y")
z = tf.multiply(x, y, name="z")
with tf.Session() as session:
print session.run(z, feed_dict={x: 2.1, y: 3.0})
2
3
4
5
6
7
8
9
用TensorBoard可视化计算图
TensorBoard是一个分析数据流图的可视化工具。这对于更好地理解机器学习模型非常有用。 使用TensorBoard,您可以深入了解不同类型的统计信息,这些统计信息通常包含有关计算图部分的参数和详细信息。深度神经网络具有大量的节点并不罕见。TensorBoard允许开发人员深入了解每个节点以及如何通过TensorFlow运行时执行计算。 现在让我们回到TensorFlow教程开始的例子,在这里我们定义了一个线性函数
y = a*x + b
。 为了从会话中记录事件,以后可以在TensorBoard中使用,TensorFlow提供了这个FileWriter
类。它可以用来创建一个事件文件来存储摘要和事件,其中构造函数接受六个参数,如下所示:
__init__(logdir, graph=None, max_queue=10, flush_secs=120, graph_def=None, filename_suffix=None)
其中logdir参数是必需的,而其他的则有默认值。图形参数将从训练程序中创建的会话对象传递。完整的示例代码如下所示:
import tensorflow as tf
x = tf.constant(-2.0, name="x", dtype=tf.float32)
a = tf.constant(5.0, name="a", dtype=tf.float32)
b = tf.constant(13.0, name="b", dtype=tf.float32)
y = tf.Variable(tf.add(tf.multiply(a, x), b))
init = tf.global_variables_initializer()
with tf.Session() as session:
merged = tf.summary.merge_all() // new
writer = tf.summary.FileWriter("logs", session.graph) // new
session.run(init)
print session.run(y)
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
我们只添加了两个新的行。我们合并在默认图中收集的所有摘要,并使用FileWriter分别将事件转储到文件中。 运行程序后,我们在目录日志中有文件,最后一步是运行tensorboard
:
tensorboard --logdir logs/
现在TensorBoard在默认端口6006上启动并运行。打开http://localhost:6006
并点击Graphs菜单项(位于页面顶部)后,您将能够看到图形,如下图所示: TensorBoard标记常量和汇总节点的特定符号,如下所述。
数学与张量流
张量是TensorFlow中的基本数据结构,它们表示数据流图中的连接边。 张量只是标识一个多维数组或列表。张量结构可以用三个参数来标识:等级,形状和类型。
- 等级:标识张量的维数。秩被称为张量的阶数或n维,其中例如秩1张量是矢量或秩2张量是矩阵。
- 形状:张量的形状是它所具有的行数和列数。
- 类型:分配给张量元素的数据类型。
为了在TensorFlow中建立一个张量,我们可以建立一个n维数组。这可以通过使用NumPy库或通过将Python n维数组转换为TensorFlow张量来轻松完成。 为了构建一维张量,我们将使用一个NumPy数组,我们将通过传递一个内置的Python列表来构造这个数组。
import numpy as np
tensor_1d = np.array([1.45, -1, 0.2, 102.1])
2
使用这种数组类似于使用内置的Python列表。主要区别在于NumPy数组还包含一些其他属性,如尺寸,形状和类型。
>> print tensor_1d
[ 1.45 -1. 0.2 102.1 ]
>> print tensor_1d[0]
1.45
>> print tensor_1d[2]
0.2
>> print tensor_1d.ndim
1
>> print tensor_1d.shape
(4,)
>> print tensor_1d.dtype
float64
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
NumPy数组可以很容易地用辅助函数convert_to_tensor转换成TensorFlow张量,这可以帮助开发人员将Python对象转换成张量对象。这个函数接受张量对象,NumPy数组,Python列表和Python标量。
tensor = tf.convert_to_tensor(tensor_1d, dtype=tf.float64)
现在,如果我们将张量绑定到TensorFlow会话,我们将能够看到我们转换的结果。
tensor = tf.convert_to_tensor(tensor_1d, dtype=tf.float64)
with tf.Session() as session:
print session.run(tensor)
print session.run(tensor[0])
print session.run(tensor[1])
2
3
4
5
6
输出:
[ 1.45 -1. 0.2 102.1 ]
1.45
-1.0
2
3
我们可以用类似的方法创建一个二维张量或矩阵:
tensor_2d = np.array(np.random.rand(4, 4), dtype='float32')
tensor_2d_1 = np.array(np.random.rand(4, 4), dtype='float32')
tensor_2d_2 = np.array(np.random.rand(4, 4), dtype='float32')
m1 = tf.convert_to_tensor(tensor_2d)
m2 = tf.convert_to_tensor(tensor_2d_1)
m3 = tf.convert_to_tensor(tensor_2d_2)
mat_product = tf.matmul(m1, m2)
mat_sum = tf.add(m2, m3)
mat_det = tf.matrix_determinant(m3)
with tf.Session() as session:
print session.run(mat_product)
print session.run(mat_sum)
print session.run(mat_det)
2
3
4
5
6
7
8
9
10
11
12
13
14
15
张量操作
在上面的例子中,我们在矢量和矩阵上引入了一些TensorFlow操作。这些操作对张量执行一定的计算。下面的表显示了这些计算。
| TensorFlow操作符 | 描述 |
| --------------- | -------- |
| [tf.add][] | X + Y |
| [tf.subtract][] | XY |
| [tf.multiply][] | X \* Y |
| [tf.div][] | x / y的 |
| [tf.mod][] | x%y |
| [tf.abs][] | | X | |
| [tf.negative][] | -X |
| [tf.sign][] | 签(x)的 |
| [tf.square][] | X \* X |
| [tf.round][] | 轮(x)的 |
| [tf.sqrt][] | SQRT(x)的 |
| [tf.pow][] | X ^ÿ |
| [tf.exp][] | E 1 X |
| [tf.log][] | 日志(X) |
| [tf.maximum][] | max(x,y) |
| [tf.minimum][] | min(x,y) |
| [tf.cos][] | COS(x)的 |
| [tf.sin][] | 的sin(x) |
上表中列出的TensorFlow操作使用张量对象,并按元素执行。所以,如果你想计算一个向量x的余弦,TensorFlow操作将对通过的张量中的每个元素进行计算。
tensor_1d = np.array([0, 0, 0])
tensor = tf.convert_to_tensor(tensor_1d, dtype=tf.float64)
with tf.Session() as session:
print session.run(tf.cos(tensor))
2
3
4
输出:
[ 1. 1. 1.]
矩阵操作
矩阵运算对于像线性回归这样的机器学习模型非常重要,因为它们经常被用在其中。TensorFlow支持所有最常见的矩阵运算,像乘法,移调,反转,计算行列式,求解线性方程组,并有更多的。 接下来,我们将解释一些矩阵操作。像线性回归一样,它们在机器学习模型中往往很重要。让我们写一些代码,将做到基本的矩阵运算像乘法,获得转置,得到了决定,乘法,溶胶,等等。 以下是调用这些操作的基本示例。
import tensorflow as tf
import numpy as np
def convert(v, t=tf.float32):
return tf.convert_to_tensor(v, dtype=t)
m1 = convert(np.array(np.random.rand(4, 4), dtype='float32'))
m2 = convert(np.array(np.random.rand(4, 4), dtype='float32'))
m3 = convert(np.array(np.random.rand(4, 4), dtype='float32'))
m4 = convert(np.array(np.random.rand(4, 4), dtype='float32'))
m5 = convert(np.array(np.random.rand(4, 4), dtype='float32'))
m_tranpose = tf.transpose(m1)
m_mul = tf.matmul(m1, m2)
m_det = tf.matrix_determinant(m3)
m_inv = tf.matrix_inverse(m4)
m_solve = tf.matrix_solve(m5, [[1], [1], [1], [1]])
with tf.Session() as session:
print session.run(m_tranpose)
print session.run(m_mul)
print session.run(m_inv)
print session.run(m_det)
print session.run(m_solve)
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
转换数据
Reduction
TensorFlow支持不同类型的缩减。简化是通过跨越这些维度执行某些操作,从张量中移除一个或多个维度的操作。当前版本的TensorFlow支持的减少列表可以在这里找到。我们将在下面的例子中展示其中的一些。
import tensorflow as tf
import numpy as np
def convert(v, t=tf.float32):
return tf.convert_to_tensor(v, dtype=t)
x = convert(
np.array(
[
(1, 2, 3),
(4, 5, 6),
(7, 8, 9)
]), tf.int32)
bool_tensor = convert([(True, False, True), (False, False, True), (True, False, False)], tf.bool)
red_sum_0 = tf.reduce_sum(x)
red_sum = tf.reduce_sum(x, axis=1)
red_prod_0 = tf.reduce_prod(x)
red_prod = tf.reduce_prod(x, axis=1)
red_min_0 = tf.reduce_min(x)
red_min = tf.reduce_min(x, axis=1)
red_max_0 = tf.reduce_max(x)
red_max = tf.reduce_max(x, axis=1)
red_mean_0 = tf.reduce_mean(x)
red_mean = tf.reduce_mean(x, axis=1)
red_bool_all_0 = tf.reduce_all(bool_tensor)
red_bool_all = tf.reduce_all(bool_tensor, axis=1)
red_bool_any_0 = tf.reduce_any(bool_tensor)
red_bool_any = tf.reduce_any(bool_tensor, axis=1)
with tf.Session() as session:
print "Reduce sum without passed axis parameter: ", session.run(red_sum_0)
print "Reduce sum with passed axis=1: ", session.run(red_sum)
print "Reduce product without passed axis parameter: ", session.run(red_prod_0)
print "Reduce product with passed axis=1: ", session.run(red_prod)
print "Reduce min without passed axis parameter: ", session.run(red_min_0)
print "Reduce min with passed axis=1: ", session.run(red_min)
print "Reduce max without passed axis parameter: ", session.run(red_max_0)
print "Reduce max with passed axis=1: ", session.run(red_max)
print "Reduce mean without passed axis parameter: ", session.run(red_mean_0)
print "Reduce mean with passed axis=1: ", session.run(red_mean)
print "Reduce bool all without passed axis parameter: ", session.run(red_bool_all_0)
print "Reduce bool all with passed axis=1: ", session.run(red_bool_all)
print "Reduce bool any without passed axis parameter: ", session.run(red_bool_any_0)
print "Reduce bool any with passed axis=1: ", session.run(red_bool_any)
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
输出:
Reduce sum without passed axis parameter: 45
Reduce sum with passed axis=1: [ 6 15 24]
Reduce product without passed axis parameter: 362880
Reduce product with passed axis=1: [ 6 120 504]
Reduce min without passed axis parameter: 1
Reduce min with passed axis=1: [1 4 7]
Reduce max without passed axis parameter: 9
Reduce max with passed axis=1: [3 6 9]
Reduce mean without passed axis parameter: 5
Reduce mean with passed axis=1: [2 5 8]
Reduce bool all without passed axis parameter: False
Reduce bool all with passed axis=1: [False False False]
Reduce bool any without passed axis parameter: True
Reduce bool any with passed axis=1: [ True True True]
2
3
4
5
6
7
8
9
10
11
12
13
14
还原算子的第一个参数是我们想要减小的张量。第二个参数是我们要执行缩减的维度的索引。该参数是可选的,如果不通过,则会沿着所有维度执行缩减。 我们可以看看reduce_sum操作。我们通过一个二维张量,并想要沿着第一维将其缩小。 在我们的情况下,由此产生的总和将是:
[1 + 2 + 3 = 6, 4 + 5 + 6 = 15, 7 + 8 + 9 = 24]
如果我们通过维度0,结果将是:
[1 + 4 + 7 = 12, 2 + 5 + 8 = 15, 3 + 6 + 9 = 18]
如果我们不通过任何轴,结果只是总和:
1 + 4 + 7 = 12, 2 + 5 + 8 = 15, 3 + 6 + 9 = 45
所有简化功能都有类似的界面,并列在TensorFlow 简化文档中。
分割
分割是一个过程,其中一个维度是将维度映射到提供的分段索引上的过程,结果元素由索引行确定。 分割实际上是在重复索引下对元素进行分组,因此,例如,在我们的例子中,我们[0, 0, 1, 2, 2]
对张量应用了分割的ID tens1
,这意味着第一个和第二个数组将在分割操作之后进行变换(在我们的情况下为总和)数组,看起来像(2, 8, 1, 0) = (2+0, 5+3, 3-2, -5+5)
。张量中的第三个元素tens1
是未触及的,因为它没有被分组到任何重复的索引中,最后的两个数组和第一组的情况相同。除总结外,TensorFlow支持产品,平均值,最大值和最小值。
import tensorflow as tf
import numpy as np
def convert(v, t=tf.float32):
return tf.convert_to_tensor(v, dtype=t)
seg_ids = tf.constant([0, 0, 1, 2, 2])
tens1 = convert(np.array([(2, 5, 3, -5), (0, 3, -2, 5), (4, 3, 5, 3), (6, 1, 4, 0), (6, 1, 4, 0)]), tf.int32)
tens2 = convert(np.array([1, 2, 3, 4, 5]), tf.int32)
seg_sum = tf.segment_sum(tens1, seg_ids)
seg_sum_1 = tf.segment_sum(tens2, seg_ids)
with tf.Session() as session:
print "Segmentation sum tens1: ", session.run(seg_sum)
print "Segmentation sum tens2: ", session.run(seg_sum_1)
Segmentation sum tens1:
[[ 2 8 1 0]
[ 4 3 5 3]
[12 2 8 0]]
Segmentation sum tens2: [3 3 9]
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
序列实用程序
序列实用程序包括如下方法:
- argmin函数,该函数返回输入张量轴上最小值的索引,
- argmax函数,该函数返回输入张量轴上最大值的索引,
- setdiff计算两个数字或字符串列表之间的差异,
- where 函数,这将返回元素从两个传递的元素x或y,这取决于传递的条件,或
- unique 功能,它将返回一维张量中的独特元素。
我们在下面演示一些执行示例:
import numpy as np
import tensorflow as tf
def convert(v, t=tf.float32):
return tf.convert_to_tensor(v, dtype=t)
x = convert(np.array([
[2, 2, 1, 3],
[4, 5, 6, -1],
[0, 1, 1, -2],
[6, 2, 3, 0]
]))
y = convert(np.array([1, 2, 5, 3, 7]))
z = convert(np.array([1, 0, 4, 6, 2]))
arg_min = tf.argmin(x, 1)
arg_max = tf.argmax(x, 1)
unique = tf.unique(y)
diff = tf.setdiff1d(y, z)
with tf.Session() as session:
print "Argmin = ", session.run(arg_min)
print "Argmax = ", session.run(arg_max)
print "Unique_values = ", session.run(unique)[0]
print "Unique_idx = ", session.run(unique)[1]
print "Setdiff_values = ", session.run(diff)[0]
print "Setdiff_idx = ", session.run(diff)[1]
print session.run(diff)[1]
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
输出:
Argmin = [2 3 3 3]
Argmax = [3 2 1 0]
Unique_values = [ 1. 2. 5. 3. 7.]
Unique_idx = [0 1 2 3 4]
Setdiff_values = [ 5. 3. 7.]
Setdiff_idx = [2 3 4]
2
3
4
5
6
用TensorFlow进行机器学习
在本节中,我们将用TensorFlow展示一个机器学习用例。第一个例子是用kNN方法分类数据的算法,第二个例子将使用线性回归算法。
k近邻
第一个算法是k-最近邻居(kNN)。这是一个监督学习算法,使用距离度量,例如欧几里德距离,对数据进行分类以训练。它是最简单的算法之一,但对分类数据仍然非常有用。这个算法的优点:
- 当训练模型足够大时,给出高精度
- 对异常值通常不敏感,我们不需要对数据做任何假设。
这个算法的缺点:
- 计算昂贵,和
- 需要大量内存,需要将新分类数据添加到所有初始训练实例中。
我们将在这个代码示例中使用的距离是欧几里得,它定义了两个点之间的距离,如下所示:
在这个公式中,
n
是空间的维数,x
是训练数据的矢量,y
是我们要分类的一个新的数据点。
import os
import numpy as np
import tensorflow as tf
ccf_train_data = "train_dataset.csv"
ccf_test_data = "test_dataset.csv"
dataset_dir = os.path.abspath(os.path.join(os.path.dirname(__file__), '../datasets'))
ccf_train_filepath = os.path.join(dataset_dir, ccf_train_data)
ccf_test_filepath = os.path.join(dataset_dir, ccf_test_data)
def load_data(filepath):
from numpy import genfromtxt
csv_data = genfromtxt(filepath, delimiter=",", skip_header=1)
data = []
labels = []
for d in csv_data:
data.append(d[:-1])
labels.append(d[-1])
return np.array(data), np.array(labels)
train_dataset, train_labels = load_data(ccf_train_filepath)
test_dataset, test_labels = load_data(ccf_test_filepath)
train_pl = tf.placeholder("float", [None, 28])
test_pl = tf.placeholder("float", [28])
knn_prediction = tf.reduce_sum(tf.abs(tf.add(train_pl, tf.negative(test_pl))), axis=1)
pred = tf.argmin(knn_prediction, 0)
with tf.Session() as tf_session:
missed = 0
for i in xrange(len(test_dataset)):
knn_index = tf_session.run(pred, feed_dict={train_pl: train_dataset, test_pl: test_dataset[i]})
print "Predicted class {} -- True class {}".format(train_labels[knn_index], test_labels[i])
if train_labels[knn_index] != test_labels[i]:
missed += 1
tf.summary.FileWriter("../samples/article/logs", tf_session.graph)
print "Missed: {} -- Total: {}".format(missed, len(test_dataset))
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
我们在上面的例子中使用的数据集是可以在Kaggle数据集部分找到的数据集。我们使用了一个包含欧洲持卡人的信用卡交易。我们使用的数据没有任何清理或过滤,根据Kaggle对这个数据集的描述,这是非常不平衡的。数据集包含31个变量:时间,V1,…,V28,数量和类别。在这个代码示例中,我们只使用V1,…,V28和Class。类别标签具有1的欺诈性交易和不具有0的交易。 代码示例主要包含前面章节介绍的内容,除了介绍加载数据集的函数外。该函数load_data(filepath)
将采用一个CSV文件作为参数,并返回一个元组,其中包含CSV中定义的数据和标签。 就在这个函数下面,我们定义了测试和训练数据的占位符。在预测模型中使用训练的数据来解析需要分类的输入数据的标签。在我们的例子中,kNN使用欧几里得距离来获得最近的标签。 错误率可以通过简单的划分来计算,当一个分类器错过了我们对这个数据集的例子总数为0.2(即分类器给我们20%的测试数据的错误的数据标签)。
线性回归
线性回归算法寻找两个变量之间的线性关系。如果我们将因变量标记为y,将自变量标记为x,那么我们试图估计函数的参数y = Wx + b
。 线性回归是应用科学领域中广泛使用的算法。该算法允许在实现中增加两个重要的机器学习概念:成本函数和梯度下降法,以找出函数的最小值。 使用这种方法实现的机器学习算法必须预测y
作为x
线性回归算法将确定值的位置的值,W
并且b
其实际上是未知的,并且是在训练过程中确定的。选择成本函数,并且通常使用均方误差,其中梯度下降是用于找到成本函数的局部最小值的优化算法。 梯度下降法只是一个局部函数最小值,但它可以用于搜索全局最小值,一旦找到一个局部最小值并随机重复这个过程,就可以随机选择一个新的起点。如果函数的极小值数目有限且尝试次数非常多,那么很有可能在某一时刻发现全局最小值。有关这种技术的更多细节,我们将在介绍部分提到的文章中留下。
import tensorflow as tf
import numpy as np
test_data_size = 2000
iterations = 10000
learn_rate = 0.005
def generate_test_values():
train_x = []
train_y = []
for _ in xrange(test_data_size):
x1 = np.random.rand()
x2 = np.random.rand()
x3 = np.random.rand()
y_f = 2 * x1 + 3 * x2 + 7 * x3 + 4
train_x.append([x1, x2, x3])
train_y.append(y_f)
return np.array(train_x), np.transpose([train_y])
x = tf.placeholder(tf.float32, [None, 3], name="x")
W = tf.Variable(tf.zeros([3, 1]), name="W")
b = tf.Variable(tf.zeros([1]), name="b")
y = tf.placeholder(tf.float32, [None, 1])
model = tf.add(tf.matmul(x, W), b)
cost = tf.reduce_mean(tf.square(y - model))
train = tf.train.GradientDescentOptimizer(learn_rate).minimize(cost)
train_dataset, train_values = generate_test_values()
init = tf.global_variables_initializer()
with tf.Session() as session:
session.run(init)
for _ in xrange(iterations):
session.run(train, feed_dict={
x: train_dataset,
y: train_values
})
print "cost = {}".format(session.run(cost, feed_dict={
x: train_dataset,
y: train_values
}))
print "W = {}".format(session.run(W))
print "b = {}".format(session.run(b))
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
输出:
cost = 3.1083032809e-05
W = [[ 1.99049103]
[ 2.9887135 ]
[ 6.98754263]]
b = [ 4.01742554]
2
3
4
5
在上面的例子中,我们有两个新的变量,我们叫cost
和train
。通过这两个变量,我们定义了一个我们想要在我们的训练模型中使用的优化器,以及我们想要最小化的函数。 在结束时,输出参数W
和b
应该是那些在定义的完全相同generate_test_values
的功能。在第17行,我们实际上定义了我们用来产生线性数据点来训练,其中的函数w1=2
,w2=3
,w3=7
和b=4
。上述例子的线性回归是使用多个独立变量的多变量。
结论
从这个TensorFlow教程中可以看到,TensorFlow是一个功能强大的框架,它使数学表达式和多维数组的工作变得轻而易举,这在机器学习中是非常必要的。它还抽象了执行数据图和缩放的复杂性。 随着时间的推移,TensorFlow已经越来越流行,现在开发人员正在使用深度学习方法来解决问题,如图像识别,视频检测,文本处理(如情感分析)等。像任何其他库一样,您可能需要一些时间来使用到TensorFlow构建的概念。而且,一旦你做到了,在文档和社区支持的帮助下,将问题表示为数据图并用TensorFlow解决问题,可以使机器学习成为一个不那么繁琐的过程。
了解基础知识
[toggle hide=“yes” title=“TensorFlow常量是如何创建的?” color=“”] 在TensorFlow中,使用常量函数创建常量,其中包含几个参数:值,dtype(数据类型),形状,名称和(verify_shape)形状验证。 [/toggle] [toggle hide=“yes” title=“什么是TensorFlow会话?” color=“”] 会话(Session)封装了TensorFlow运行时的控制和状态。没有参数的会话将使用在当前会话中创建的默认图形,否则会话类接受在该会话中使用的图形参数来执行。 [/toggle] [toggle hide=“yes” title=“什么是TensorBoard?” color=“”] TensorBoard是一个分析数据流图的可视化工具。这对于更好地理解机器学习模型非常有用。 [/toggle]
扩展资料
关于作者 Dino拥有超过五年的软件开发经验。在过去的两年里,他一直从事Java和相关技术方面的工作,主要是使用NoSQL技术实现大数据解决方案,并实施REST服务。他还拥有.NET和PCI DSS安全标准方面的经验,以及使用Python和已有框架的REST解决方案。
除特别注明外,本站所有文章均为 windcoder 原创,转载请注明出处来自: tensorflowrumenyipianjiqixuexijiaocheng
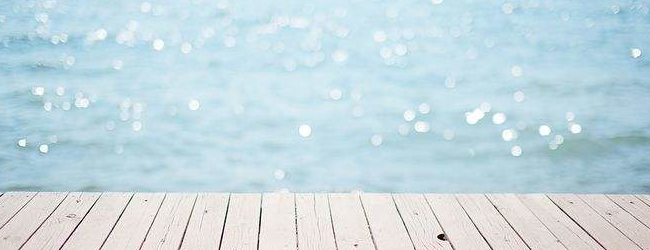
暂无数据