在数组中查找次大值,并与最后一个元素交换—C语言
/***************************************************
作业要求:
在数组中查找次大值,并与最后一个元素交换
完成日期:
2013年9月3日
***************************************************/
#include <stdio.h>
// 函数原型
int findSecondMaxValueInArray(int a[], int n);
// main函数
int main(void)
{
int a[8] = {2, 5, 1, 3, 2, 3, 4, 6}; // 定义数组
int index; // 待求次大值元素下标
int tmp; // 临时变量,用来交换数组
// 求数组中次大值元素下标
index = findSecondMaxValueInArray(a, 8);
// printf("%dn", index);
// 次大值与数组最后一个元素交换
tmp = a[index];
a[index] = a[7];
a[7] = tmp;
// 输出数组……
return 0;
}
/****************************************************
函数功能:
在数组中查找次大值元素
算法思想:
(1) 设置两个指针(下标)初始值均为0(指向数组第1个元素);
(2) 遍历数组,若当前元素大于最大值,修改最大值下标为当前元素;
修改次大值下标为原来最大值下标;
(3) 若当前元素不大于最大值,但大于次大值,则修改次大值下标为
当前元素;
(4) 数组遍历结束后,次大值下标即为所求。
函数参数:
int a[] 待查找元素的数组
int n 数组中元素个数
返回值:
返回次大值元素在数组中的下标
时间复杂度:
O(n):其中n表示数组中元素个数
空间复杂度:
O(1):借助了三个辅助变量i、max1、max2实现
*****************************************************/
int findSecondMaxValueInArray(int a[], int n)
{
int i; // 数组元素索引(下标)
int max1 = 0; // 最大值元素下标
int max2 = 0; // 次大值元素下标
for (i = 0; i < n; ++i)
{
if (a[max1] < a[i])
{
max2 = max1; // 原来最大值为新的次大值
max1 = i; // 当前元素为新的最大值
}
else if (a[max2] < a[i])
{
// 若新的最大值没有出现,但是数组中元素大于次大值
max2 = i;
}
}
// 返回次大值下标
return max2;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
预览
除特别注明外,本站所有文章均为 windcoder 原创,转载请注明出处来自: zai-shu-zu-zhong-cha-zhao-ci-da-zhi-bing-yu-zui-hou-yi-ge-yuan-su-jiao-huan-c-yu-yan
Loading comments...
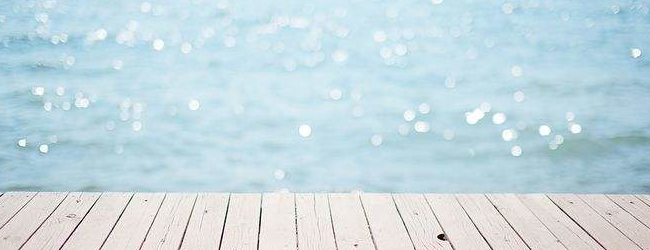
预览
暂无数据